Learn Programming: Variables and Constants
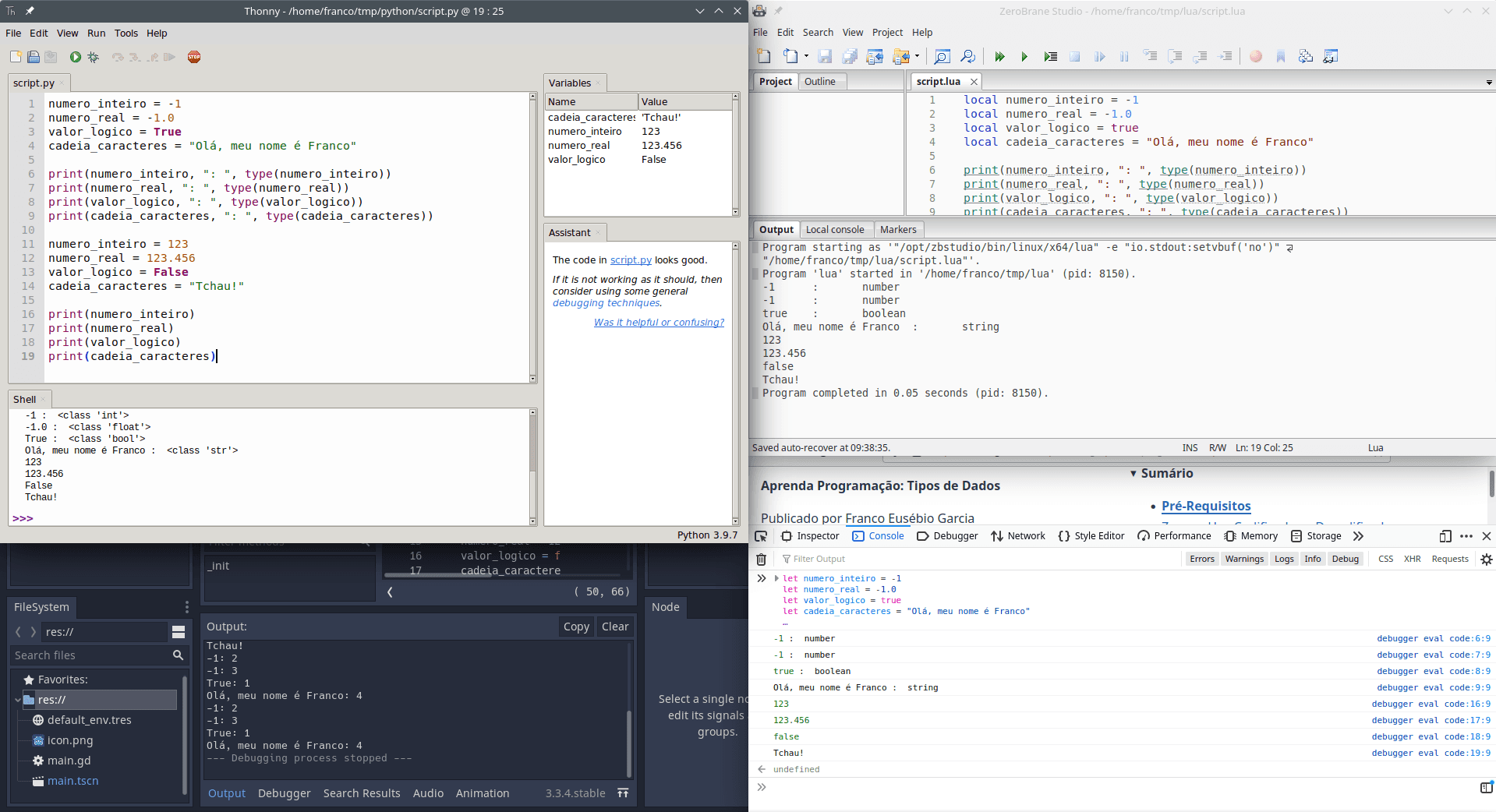
Image credits: Image created by the author using the program Spectacle.
Requirements
In the introduction to development environments, I have mentioned Python, Lua and JavaScript as good choices of programming languages for beginners. Later, I have commented about GDScript as an option for people who want to program digital games or simulations. For the introductory programming activities, you will need, at least, a development environment configured for one of the previous languages.
If you wish to try programming without configuring an environment, you can use of the online editors that I have created:
However, they do not provide all features offered by interpreters for the languages. Thus, sooner or later, you will need to set up a development environment. If you need to configure one, you can refer to the following resources.
Thus, if you have an Integrated Development Environment (IDE), or a combination of text editor and an interpreter, you are ready to start. The following example assumes that you know how to run code in your chosen language, as presented in the configuration pages.
If you want to use another language, the introduction provides links for configure development environments for the C, C++, Java, LISP, Prolog, and SQL (with SQLite) languages. In many languages, it suffices to follow the models from the experimentation section to modify syntax, commands and functions from the code blocks. C and C++ are exceptions, for they require pointers access the memory.
Memory
In the last topic, it was defined what are data types Data types abstract encoded values. In computer architectures, values must exist somewhere. This somewhere is called memory.
Programming activities commonly use two types of memory: primary memory and secondary memory.
Primary Memory
In programming, primary memory is, often, a synonym random-access memory (RAM). The RAM is a memory providing fast access, small to medium quantities (depending on the device, it can range from Kilobytes to Gigabytes), and volatile. A volatile memory is the one that does not persist data when power stops being provided to it. In other words, the contents are lost every time that a device is turned off. Whether it is by an action of a person, whether it is due to a problem (such as power outage or an electronic component failure), all data are lost when power ends.
If you had, at any time, lost data due to a program crash or because of power outage, the reason is that the data was only stored in primary memory. This is why it is important to use the features to save data (such as documents). The act of saving copy data from the primary memory to the secondary memory, used as persistent storage. Secondary memory is persistent, which is the antonym of volatile.
There exists other types of primary memory. For instance, register are the memory used by the processor to perform instructions. Register share many of the characteristics of primary memory, though they are faster and with an even smaller capacity (ranging from Kilobytes to a few Megabytes). Depending on the computer architecture, it is possible to consider registers as primary memory or as a category of their own.
However, for practical purposes, the term primary memory is usually related to using the RAM. Except in languages that allow programming in a very low-level (such as C and C++) or in machine languages (such as assembly), the programming language abstract the use of registers, enabling only variables for coding at a higher abstraction level.
Secondary Memory
Secondary memory is a memory that is auxiliary to the primary one. It is, often, the one that common sense calls "memory" : the memory used for persistent data storage.
The secondary memory is slower than the primary, though it provides greater storage capacity (for instance, in the order of Terabytes) and it is persistent. As previously commented, persistent memory is the opposite of volatile memory. When data is saved in persistent memory, they are persisted (that is, they keep existing) even when there is no power. This is done using technologies such as electromagnetism.
When it is said that secondary memory is slower than primary memory, it should be noted that it really is slower. Access, read and write times in secondary memory is much greater than in primary memory. Depending on the hardware and the quantity of data, the ratio can be in the order of hundreds, thousands or millions of times slower.
That is the reason why programs operate on primary memory instead of secondary memory. The use of secondary memory is, often, a bottleneck for the performance of a program. This is why, when a program is first opened, it is usually necessary to wait some time before being able to use it. This time results from a transfer of data from the secondary to the primary memory, containing required data to use the program. After they are loaded, the program tends to run faster.
Examples of secondary memory include hard disk drives (HDD), solid slate drives (SSD), flash memory (such as USB sticks), compact disks (CDs), and digital video disks (DVDs). Depending on your age, perhaps you have used floppy disks. They are another example of secondary memory.
In programming, secondary memory is used with abstraction such as files or databases. For instance, in the environment configuration for SQLite as an example of SQL, we created and saved data in a SQLite database.
The use of secondary memory is slightly more complex than primary memory. It will be described in the future, after the introduction of other programming resources.
Variables
Programming in Assembly uses registers. Programming in programming languages use variables.
Variables can be thought as little boxes that store data. Each little box has a capacity and a memory address.
The capacity is measure in bytes, and corresponds to the size of the data type that it can store. The address is the place where the variable exists in the RAM.
In a computer, the little boxes of variables exist in the RAM. You can imagine the RAM as a shelf or cabinet with lined up little boxes.
When a program must access (read or write) the value of a variable, it accesses the memory address and performs the operation (read or write). Read data are stored in register for use.
Resuming the metaphor of little boxes and shelves, it is like if a computer picked up the little box to keep it at an easier to use place (the register). As the number of registers is limited, the program must choose which values will be in registers and which will be in the RAM (or even in secondary memory). Values are loaded as they are needed.
If you want, you can think of the secondary memory as a warehouse. It is larger, though it requires more time to fetch or store a little box inside it.
It is still possible to enhance the metaphor. Besides the secondary memory, it is possible to think about external storage or even remote one (for instance, files from the Internet or in the "cloud"). The more distant the data and the slower the speed for accessing it, greater will be the time required to retrieve the data.
Variables in Programming Languages
In higher level programming languages, the compiler or interpreter is responsible to manage the data stored in registers or RAM. As a programmer, your task is to define a variable and use it whenever you need it.
To do this, you will perform two main operations: declaration and assignment. Besides, you normally will need to define scopes to use variables.
Declaration
Before using a variable, it must be declared. In general, a declaration maps a name chosen by the programmer to a memory address in the computer. Instead of writing a binary or decimal value to access the address, the name is used whenever the address must be accessed.
As a curiosity, some programming languages map a name to a value instead of an address. This can happen, for instance, in Python. In practice, the use of the variable is normally identical to languages that map addresses to names.
The generic term the chosen name of a variable is identifier.
Programming languages define rules to designate valid characters for identifier.
In general, an identifier must start with a letter or an underscore (underline, _
).
The identifier cannot have spaces or special symbols.
After the first character, the name accepts numbers.
There are languages that impose additional restrictions.
For instance, in Prolog, an identifier for a variable must start with an upper case letter.
Others can impose fewer restrictions.
For instance, LISP allow using some characters as hyphens (-
) in variable names.
Type Declaration
In the metaphor of little boxes, the declaration serves as a label that combines a name to a box with the required size to store values of the desired type.
In statically typed languages, the declaration must explicit the desired type for a variable. In dynamically typed languages, the compiler or interpreter infers the type of a variable using the value that was assigned to it.
Some programming languages employ a hybrid approach, in which the type declaration is optional. If a type is provided, it is used. Otherwise, the interpreter or compiler will infer based on the stored value.
It is interesting to declare types to avoid ambiguities about the type of a variable or incorrect inferences from the compiler or interpreter. It also allows creating variables with the exact amount of memory required to store values of the given type.
In languages with type inference, the declaration of integer numbers and real numbers require attention.
0
is an integer number, though 0.0
is a real number.
When an exact value for a real number must be declared, it is important to add the .0
after the value.
For instance, -123456.0
or 7.0
.
If the written value is either -123456
or 7
, the variables will be integer types.
Styles for Identifiers
There are some styles to write names of variables. Some of the most popular ones include:
PascalCase
, in which the first letter of each word starts with an upper case letter;snake_case
, in which an underscore separates words;camelCase
, in which the first letter is lower case, though the first letter of every following word in a same variable name is upper case;kebab-case
, in which a hyphen separates words.
Except if a programming language imposes restrictions, you can choose the style that you prefer. Although it is also possible to mix them, I would recommend choosing a style and stick to it in a given project, to define a standard.
Naming
Except reserved words and words that do not respect naming rules, you can choose any names that you want to your variables.
There are people who like short variable names, such as x
or i
.
Although they are certainly practical to write, code is usually read more times than written.
Thus, my recommendation is that you try to choose representative names for your variables, instead of generic ones.
For instance, n
or full_name
can be variables of the type string
to store a complete name.
The second option, however, allow identifying what is stored with greater clarity.
There are also people who like to add information about the type in the name of a variable. One of the most extreme examples is the Hungarian notation. Although they can be useful, IDEs commonly provide tips about the type of a variable (for instance, when the mouse or keyboard cursor hovers on the name of a variable), which can make the notation redundant. However, if the data type is important to a given problem (for instance, problems of precision in real numbers), it can be interesting to add it to the variable as an alert or reminder.
Assignment
To read the value of a variable, it is usually enough to use its name. To modify the value of a variable, an assignment is performed.
An assignment is that the operation to designate a value to a variable. In the little box metaphor, the assignment is the process that changes the value stored inside the box. The previous value ceases to exist, and the variable assumes the new provided value.
The name or symbol of the assignment operator varies according to programming language.
Common options are the use of an equal sign (=
), two points followed by an equal (:=
), or a combination of less than and hyphen (<-
), symbolizing an arrow.
For example, my_variable = 1
, my_variable := 1
or my_variable <- 1
could mean that the value 1
was stored in the variable my_variable
.
When the value is accessed using the name my_variable
, the returned value will be 1
.
Initialization
The first value assignment to a variable has a special name: initialization. Many programming languages allow to initialize a value directly in the variable declaration, which I recommend doing.
There are programming languages that initialize variables with a default value, such as a value considered as zero.
Normally, the values are zero for numbers (0
and 0.0
), false
for logic values, and the empty string (""
) for strings.
However, that not every language do so.
In languages that do not set a default value, the initial value will be unknown (also known as junk). In simple terms, whatever data that were in the memory before the declaration can serve as the initial value of the variable.
Thus, never assume the initial value for a variable before consulting the language's documentation to discover what is the default behavior. In general, it is a good practice to initialize variables in your program with appropriate initial values.
Copy of Variables
If you assign the value of a variable to another, the value of the first is copied to the second.
A copy can be a shallow copy or a deep copy, depending on the type of the variable and the programming language. Deep copies duplicate values, providing separate values for each variable. In other words, a subsequent change to one of the variables does not affect the other.
Shallow copies are performed using references. Instead of duplicates, the values are shared. In other words, a subsequent change of value in one of the variables will also affect the other one.
For primitive data types, the copies are generally deep, resulting in duplicated values.
Scope
A scope is a region on which a name or value is valid. In programming, two scopes are normally used: global scope and local scope. There other scopes (such as static scopes), though they are more advanced.
A name defined in the global scope is valid in any part of the program. A name defined in the local scope is only valid inside a block with specific beginning and end.
In general, the local scope should be preferred whenever possible.
This happens because identifiers must be unique.
For instance, if you declare a variable called x
, no other variable in a same scope can also be named x
.
In other words, if the scope of x
is global, the program cannot admit any other variable called x
.
Technically, some programming languages define rules that allow hiding more external scopes from more internal ones. This is often called shadowing. In such languages, the chosen value of a variable will be the one belonging to the most internal scope whenever a name conflict happens.
At this time, the choice of scopes does not make difference. However, after introducing subroutines (functions, procedures and methods), a misuse of the global scope can result in (potentially hard to debug) errors. Thus, again, try to create a habit of using the local scope whenever possible.
There are valid, more advanced uses for the global scope, such as sharing memory. Yet, they can often be avoided with the creation of abstractions using local scopes.
State
State is a term related to the use of variables. State means something that behavior or operation can vary according to the currently stored values.
A program with state can remember what happened previously, because the past values are saved in variables. Programs with state are called stateful. The result of stateful programs can vary accordingly to inputs and the current state.
For an analogy, one can think of a person in two distinct days. In a day that she/he is happy, a greeting can result into a positive reply. In a day that she/he is really angry, the greeting can be ignored or result into a negative reply. In both cases, the input was the same (the greeting), though the answer varied according the internal state of the person.
Another example could the person reacting differently for greetings from people she/he know or do not know. The existence of state allows the person to remember of past interactions with other people.
A program without state is not, necessarily, a program without variables, but a program which internal variables to not interfere in the computation in a way that changes the results; it does not register what happened in the past. A program without state is called stateless. The result of a stateless program depends only on its inputs.
For the previous example, the person would react the exact same way for every greeting. She/he would not remember of any other person; every greeting would be like the very first interaction.
Variables in Programming
It is simple to use variables in programming languages. The concepts may seem harder than they really are. It is time for practical examples.
Flowcharts: Flowgorithm
Variables in Flowgorithm uses both the concepts of declaration and assignment.
To create a new variable, right-click an arrow, then choose the Declare block. Double-click the new block. Choose a name and a type for the variable. Variable names' in Flowgorithm must start with a letter; after the first letter, the name can only have letters and numbers. At this moment, keep the option Array disabled. Then confirm the creation in OK.
To perform an assignment, right-click an arrow (it must be after the variable declaration) and choose the option Assign.
Type the name of the variable, the desired value and confirm.
Strings must be written between double quotes; logic values must be either true
or false
.
To write a value in the console, create an output block using Output and type the name of the variable.
If you want to combine text and the value of the variable, you can write the message between double quotes, add an ampersand symbol (&
) to concatenate values (which means writing one text after the first one), and type the name of the variable after it.
For instance, for a variable called integerNumber
, you could write "Value: " & integerNumber
.
To continue the text, it would suffice to perform another concatenation.
For instance, "Value: " & integerNumber & ". Text continues here."
.
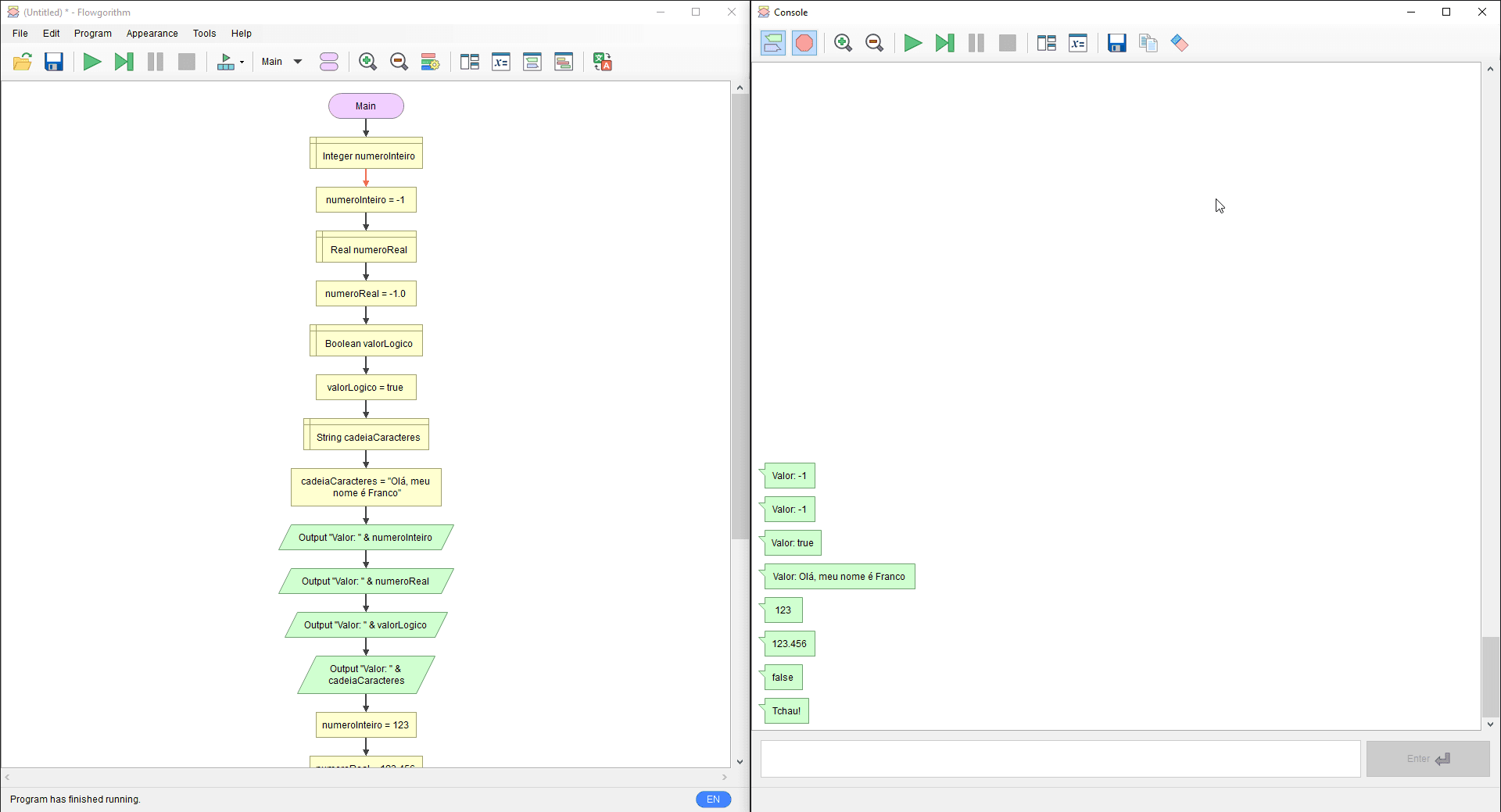
In the image, the identifiers are in Portuguese.
numeroInteiro
means integerNumber
; numeroReal
means realNumber
; valorLogico
means logicValue
; cadeiaCaracteres
means string
.
The message Olá, meu nome é Franco
means Hello, my name is Franco
.
As it can be hard to view the previous image, the next one shows a scaled flowchart.

Flowgorithm also can generate and allows visualizing implementations of the flowchart in different programming languages. To do this, click on Tools, then on Source Code Viewer. The default option uses a kind of pseudocode (Auto Pseudocode) You can change it to a programming language available in the list, to compare a language's syntax with the syntax from others.
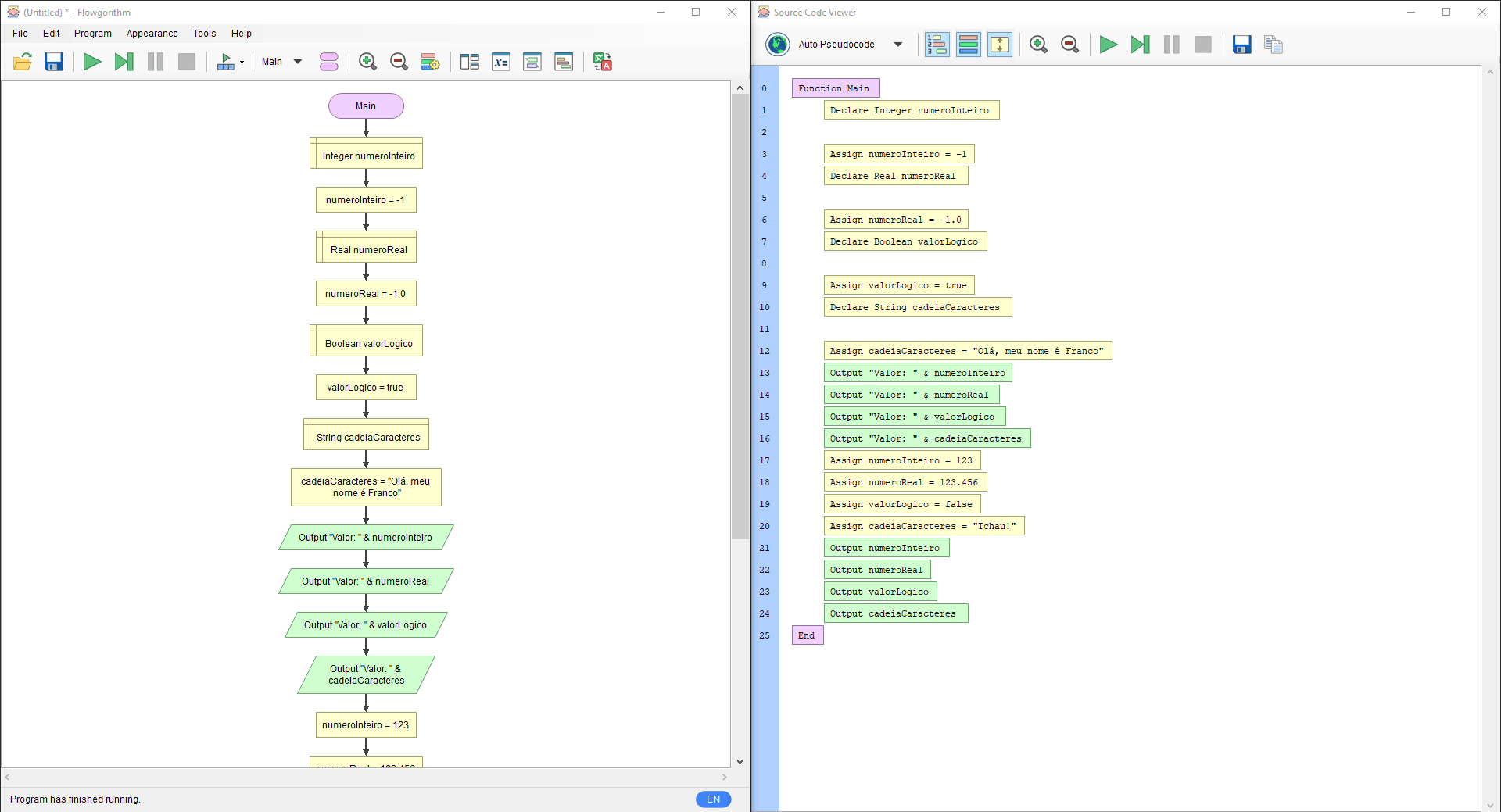
It is interesting to note that the colors of the illustrated code match the colors of the flowchart. Therefore, you can compare the structure of the program in each representation.
Visual Programming Language: Scratch
In Scratch, options to use variables are provided in the Variables side menu.
To declare a variable, click on Make a Variable.
Choose a name (for instance, my_variable
), and, optimally, a sprite.
To start, you can keep the default option (For all sprites).
Next, confirm,
An ellipse (a shape with rounded corners) will appear with the name of the variable. Whenever you want to use it, you will have to select it.
To make an assignment, you will use the block set variable name
to value
.
You can click on variable name
to choose any declared variable that you wish.
In value
, you can define the value of a variable.
If you wish to always show a variable's value in the program output pane, mark the checkbox next to the ellipse with the variable.
To write the value of a variable, add a block say Hello!.
Next, change Hello!
with the block with the desired variable.
To do this, drag the ellipse with the variable into Hello
and drop it.
To make it easier to view values, it is convenient to set a time interval between blocks.
To do this, access Control and choose the block Wait 1
seconds.
Another option is using the block Say Hello!
for 2
seconds.
You can change the value 1
or 2
by another other number that you want.
The number can even be stored in a numerical variable.
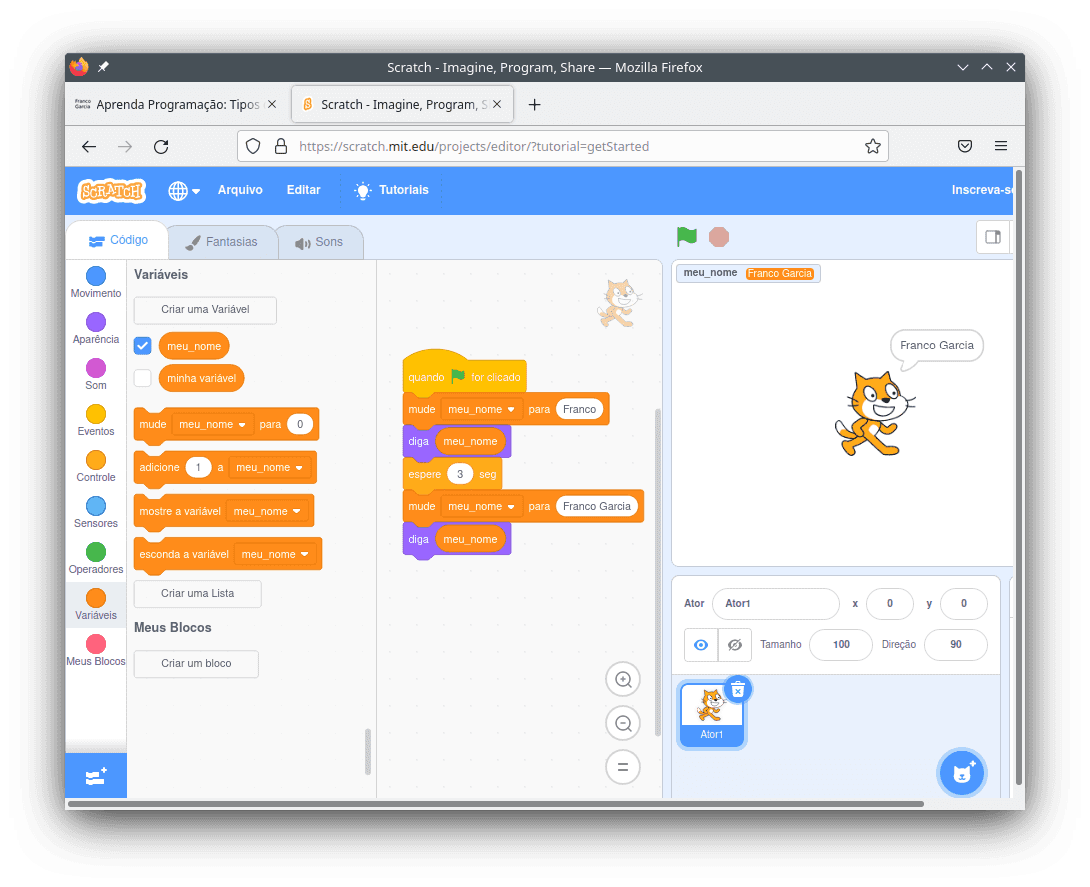
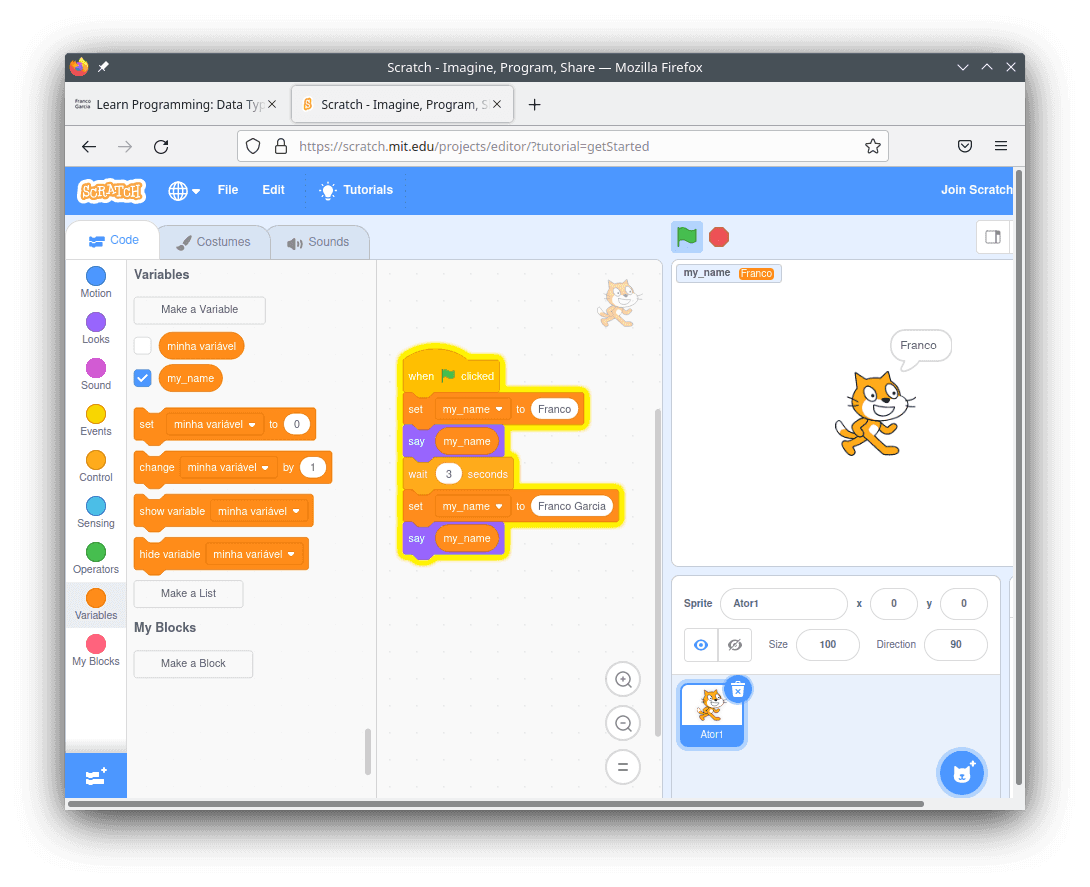
To change the name of a variable or delete it, you can right-click in an ellipse with a variable and choose the corresponding option.
You should note that Scratch does not provide a type for logic values.
If you want to use them, you can use True
or False
as text, or 1
for True
and 0
for False
.
A problem is that, later, it will be hard without proper logic values.
An alternative is to use the block Not (empty) as True
, and the block And (empty) as False
.
When empty, the previous blocks return, respectively, true
and false
, serving the purposes (although unnecessarily obscure).
Finally, if you want to add a comment to a block, you can right-click a block and choose the option Add Comment.
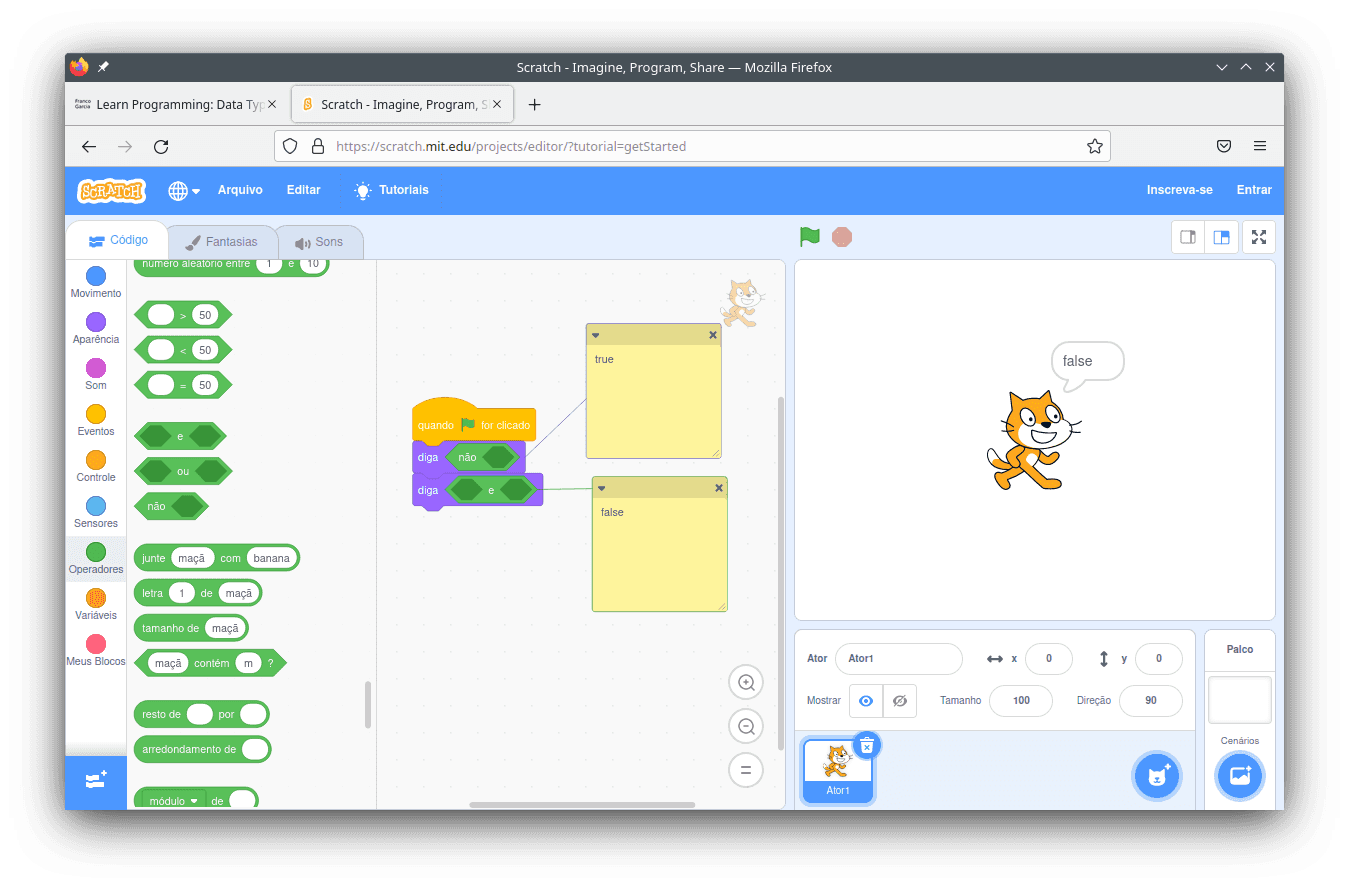
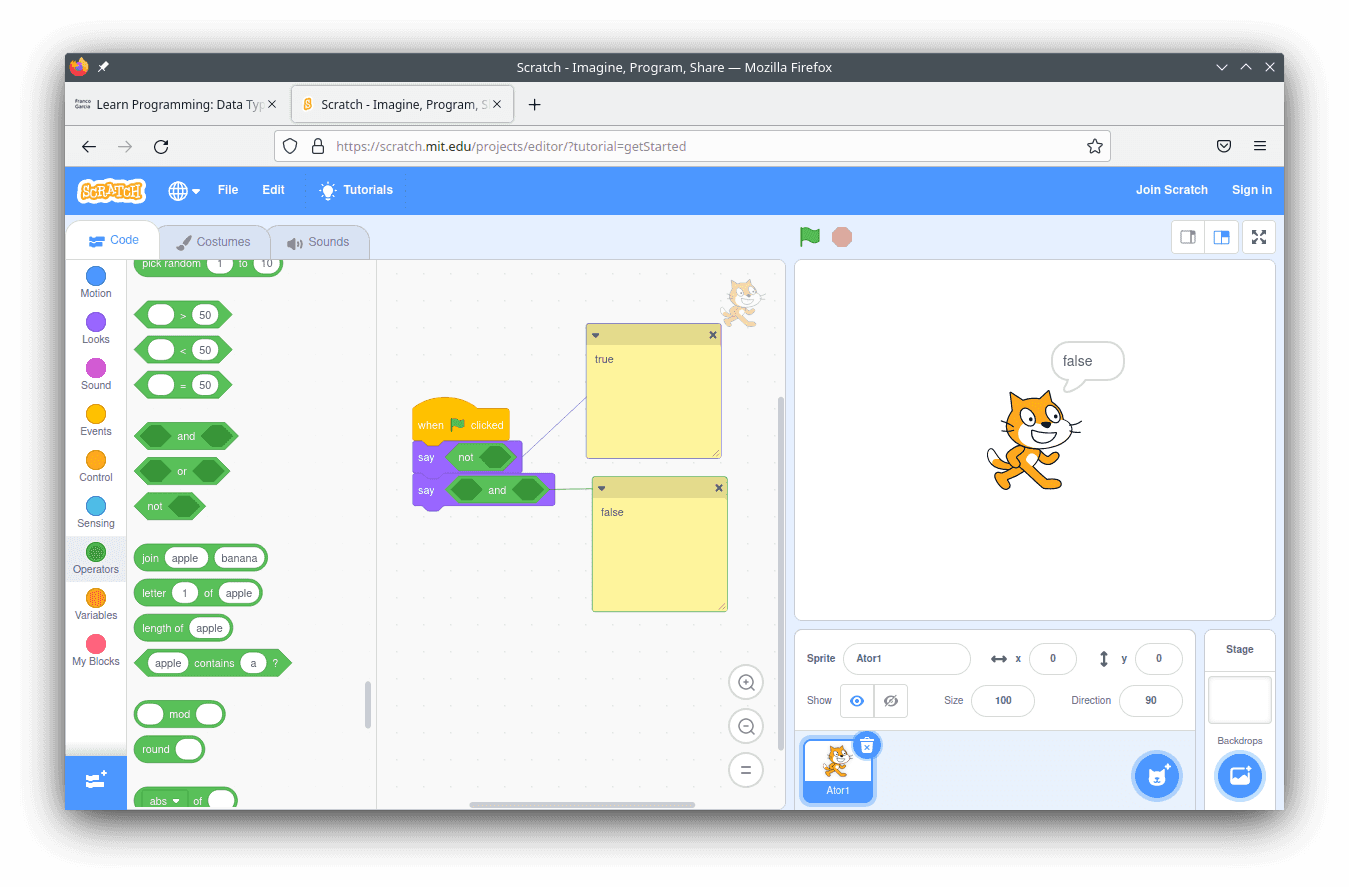
The comment adds a note to the selected block. You can write any text that you want.
Text Programming Languages: JavaScript, Python, Lua, GDScript and C++
JavaScript, Python, Lua and GDScript are languages with dynamic typing, that is, on which the interpreter can infer the type of variable after the initialization or assignment of a value.
The previous four languages define the type of the variable according the provided value. In other words, if a value of a different type is assigned, the type of the variable will change to match the new value.
It is not every programming language that allows such change. In languages such as C, C++ and Java, it is usually not possible to assign a value that does not correspond to the type of the variable.
Personally, I like to follow the convention that every variable has a type, and that it should never be changed for the same variable. This makes it easier to understand source code, because the type will always be the one proposed in the initialization.
GDScript optionally allows to declare a desired type to a variable. If provided, it will be adopted to the variable. Python also allows type suggestion in some circumstances. JavaScript has an alternative implementation called TypeScript, that add support for explicit types.
As the declarations for JavaScript, Python, Lua and GDScript will be similar, the following example also declares variables in C++.
Furthermore, the GDScript version provides examples of the optional typing after the standard declaration.
This is performed in two ways: with the type suggestion, and with the use of a special operator, :=
, that adopts the type of the initialized value as the type of the variable.
When you are programming, you can choose any of the tree options to declare the variables of your program.
let integer_number = -1
let real_number = -1.0
let logic_value = true
let string_with_message = "Hello, my name is Franco"
console.log(integer_number, ": ", typeof(integer_number))
console.log(real_number, ": ", typeof(real_number))
console.log(logic_value, ": ", typeof(logic_value))
console.log(string_with_message, ": ", typeof(cadeia_caracteres))
integer_number = 123
real_number = 123.456
logic_value = false
string_with_message = "Tchau!"
console.log(integer_number)
console.log(real_number)
console.log(logic_value)
console.log(string_with_message)
integer_number = -1
real_number = -1.0
logic_value = True
string_with_message = "Hello, my name is Franco"
print(integer_number, ": ", type(integer_number))
print(real_number, ": ", type(real_number))
print(logic_value, ": ", type(logic_value))
print(string_with_message, ": ", type(cadeia_caracteres))
integer_number = 123
real_number = 123.456
logic_value = False
string_with_message = "Tchau!"
print(integer_number)
print(real_number)
print(logic_value)
print(string_with_message)
local integer_number = -1
local real_number = -1.0
local logic_value = true
local string_with_message = "Hello, my name is Franco"
print(integer_number, ": ", type(integer_number))
print(real_number, ": ", type(real_number))
print(logic_value, ": ", type(logic_value))
print(string_with_message, ": ", type(cadeia_caracteres))
integer_number = 123
real_number = 123.456
logic_value = false
string_with_message = "Tchau!"
print(integer_number)
print(real_number)
print(logic_value)
print(string_with_message)
extends Node
func _init():
var integer_number = -1
var real_number = -1.0
var logic_value = true
var string_with_message = "Hello, my name is Franco"
print(integer_number, ": ", typeof(integer_number))
print(real_number, ": ", typeof(real_number))
print(logic_value, ": ", typeof(logic_value))
print(string_with_message, ": ", typeof(cadeia_caracteres))
integer_number = 123
real_number = 123.456
logic_value = false
string_with_message = "Tchau!"
print(integer_number)
print(real_number)
print(logic_value)
print(string_with_message)
################################################################
# With explict type. #
################################################################
var integer_number_2: int = -1
var real_number_2: float = -1.0
var logic_value_2: bool = true
var string_with_message_2: String = "Hello, my name is Franco"
print(integer_number_2, ": ", typeof(integer_number_2))
print(real_number_2, ": ", typeof(real_number_2))
print(logic_value_2, ": ", typeof(logic_value_2))
print(string_with_message_2, ": ", typeof(cadeia_caracteres_2))
################################################################
# With a fixed type based in the first assignment. #
################################################################
var integer_number_3 := -1
var real_number_3 := -1.0
var logic_value_3 := true
var string_with_message_3 := "Hello, my name is Franco"
print(integer_number_3, ": ", typeof(integer_number_3))
print(real_number_3, ": ", typeof(real_number_3))
print(logic_value_3, ": ", typeof(logic_value_3))
print(string_with_message_3, ": ", typeof(cadeia_caracteres_3))
#include <iostream>
#include <string>
int main()
{
int integer_number = -1;
double real_number = -1.0;
bool logic_value = true;
std::string string_with_message = "Hello, my name is Franco";
std::cout << integer_number << std::endl;
std::cout << real_number << std::endl;
std::cout << logic_value << std::endl;
std::cout << string_with_message << std::endl;
integer_number = 123;
real_number = 123.456;
logic_value = false;
string_with_message = "Tchau!";
std::cout << integer_number << std::endl;
std::cout << real_number << std::endl;
std::cout << logic_value << std::endl;
std::cout << string_with_message << std::endl;
return 0;
}
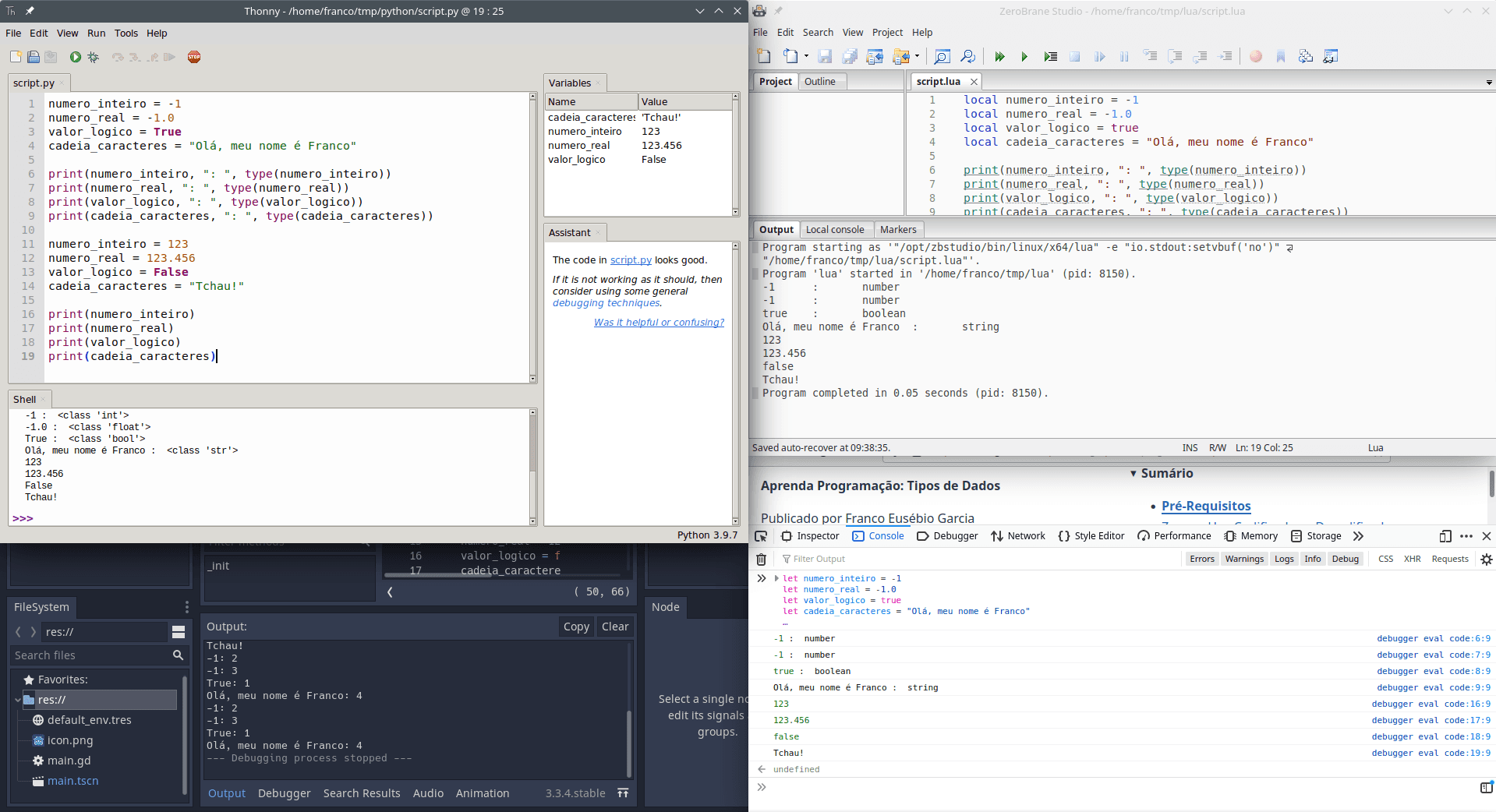
Some notes for the examples:
All variables were initialized with a value during the declaration;
The value of a variable can be changed after the declaration. Almost every programming languages allow to redefine the value of variables, though there are exceptions. For instance, logic languages, such as Prolog, can define unification instead of assignment, preventing changes to a value of a variable after unification.
In Lua,
local
allows the declaration of local variables. The same applies to JavaScript usinglet
. If the reserved word is omitted, the variable will be global. JavaScript also providesvar
for variable declaration, resulting in function scope, which is different from scope conventions for blocks used in many other programming languages. Thus, it is usually preferred to uselet
.In GDScript and C++, the declaration of variables inside
_init()
andmain()
, respectively, result in local variables. If the variables are declared outside the functions, they would be global variables.In Python, variables are global. To declare local variables, it would be necessary to add a function, such as
main()
.def main(): integer_number = -1 real_number = -1.0 logic_value = True string_with_message = "Hello, my name is Franco" print(integer_number, ": ", type(integer_number)) print(real_number, ": ", type(real_number)) print(logic_value, ": ", type(logic_value)) print(string_with_message, ": ", type(cadeia_caracteres)) integer_number = 123 real_number = 123.456 logic_value = False string_with_message = "Tchau!" print(integer_number) print(real_number) print(logic_value) print(string_with_message) if (__name__ == "__main__"): main()
Variables declared inside functions will be local variables. This is the same rules used by GDScript and C++. It also applies to many other programming languages. Exceptions include languages such as Lua and JavaScript, that provide special reserved words to declare local variables.
Variable values are, as the name suggest, variable and passable of modifications. To declare values that cannot be changed after the first assignment (that is, after the initialization), there are programming languages that allow the definition of constants.
On the other hand, many programming languages do not allow a redeclaration of a variable in a same scope. As this may happen in the JavaScript of this material, it can be useful to force the creation of a new scope using curly brackets.
{
let x = 1
console.log(x)
}
This features is convenient if an example results in a error stating a variable redeclaration.
Copies
Assignments can be performed from a variable to another. In this case, the value to the left of the assignment operator will receive the resulting value from the right of the operator.
let x = 123
let y = x
console.log(x, y)
x = 456
console.log(x, y)
x = y
console.log(x, y)
x = 123
y = x
print(x, y)
x = 456
print(x, y)
x = y
print(x, y)
local x = 123
local y = x
print(x, y)
x = 456
print(x, y)
x = y
print(x, y)
extends Node
func _init():
var x = 123
var y = x
print(x, y)
x = 456
print(x, y)
x = y
print(x, y)
#include <iostream>
#include <string>
int main()
{
int x = 123;
int y = x;
std::cout << x << " " << y << std::endl;
x = 456;
std::cout << x << " " << y << std::endl;
x = y;
std::cout << x << " " << y << std::endl;
return 0;
}
Inspecting Variables Using REPL
It is convenient to highlight a practical feature for programming using Read, Evaluate, Print, Loop (REPL). After declaring a variable, many REPLs allow one to inspect a stored value by typing the name of the variable as a command.
>>> let x = "Franco"undefined
>>> x"Franco"
js78js> let x = "Franco"js> x"Franco"
nodeWelcome to Node.js v16.11.1.
Type ".help" for more information.
> let x = "Franco"undefined
> x'Franco'
pythonPython 3.9.7 (default, Oct 10 2021, 15:13:22)
[GCC 11.1.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> x = "Franco">>> x'Franco'
luaLua 5.4.3 Copyright (C) 1994-2021 Lua.org, PUC-Rio
> x = "Franco"> xFranco
In graphical environments, some IDEs provide panels with debuggers or options to visualize variables.
Thonny
(Python), ZeroBrane Studio
(Lua), and Godot Engine (GDScript) provide embedded debuggers that can inspect variables.
Constants
Variables are values that can be modified. Constants are immutable values once initialized. An attempt to redefine the value of a constant results in error (in most programming languages, though there are exceptions).
There are some benefits provided by using a constant:
- It can make it easier to read and interpret a value, avoiding the so called
magic numbers
(arbitrary values with a value that is not immediately understood); - It allows to redefine all occurrences of its value in a project in a project in a simple and safe way. Provided that all occurrences use the name of the constant, it suffices to change the value defined in the constant declaration;
- In programming languages in which the redefinition of values for constants result in errors, it avoids accidental modification of values that should have been unalterable.
Not all languages allow the definition of constants. In languages that do not permit the creation, an alternative is to define a unique style for naming constants and ensuring that the value is never reassigned after the initialization of a variable adopted as a constant. Although it is not the ideal situation, it is a possible alternative.
Styles for Constants
It is usual to choose a style to name constants in a way that make it simple to identify them as such in the source code of a program.
Common choices include writing all letters in upper case, or adopt an arbitrary prefix, such as k
or k_
.
For instance, CONSTANT_NAME
, k_constant_name
or kConstantName
.
Text Programming Languages: JavaScript, Python, Lua, GDScript and C++
JavaScript, GDScript and C++ allow the definition of constants.
Lua allows the creation of constants since version 5.4; if you are using an older version, you will be unable to <const>
to define constants.
Python has a proposal to incorporate optional constants to the language.
The following examples illustrate how to use constants in each previous language.
const INTEGER_NUMBER = -1
const REAL_NUMBER = -1.0
const LOGIC_VALUE = true
const STRING_WITH_MESSAGE = "Hello, my name is Franco"
console.log(INTEGER_NUMBER, ": ", typeof(INTEGER_NUMBER))
console.log(REAL_NUMBER, ": ", typeof(REAL_NUMBER))
console.log(LOGIC_VALUE, ": ", typeof(LOGIC_VALUE))
console.log(STRING_WITH_MESSAGE, ": ", typeof(CADEIA_CARACTERES))
from typing import Final
INTEGER_NUMBER: Final = -1
REAL_NUMBER: Final = -1.0
LOGIC_VALUE: Final = True
STRING_WITH_MESSAGE: Final = "Hello, my name is Franco"
print(INTEGER_NUMBER, ": ", type(INTEGER_NUMBER))
print(REAL_NUMBER, ": ", type(REAL_NUMBER))
print(LOGIC_VALUE, ": ", type(LOGIC_VALUE))
print(STRING_WITH_MESSAGE, ": ", type(CADEIA_CARACTERES))
local INTEGER_NUMBER <const> = -1
local REAL_NUMBER <const> = -1.0
local LOGIC_VALUE <const> = true
local STRING_WITH_MESSAGE <const> = "Hello, my name is Franco"
print(INTEGER_NUMBER, ": ", type(INTEGER_NUMBER))
print(REAL_NUMBER, ": ", type(REAL_NUMBER))
print(LOGIC_VALUE, ": ", type(LOGIC_VALUE))
print(STRING_WITH_MESSAGE, ": ", type(CADEIA_CARACTERES))
extends Node
const INTEGER_NUMBER = -1
const REAL_NUMBER = -1.0
const LOGIC_VALUE = true
const STRING_WITH_MESSAGE = "Hello, my name is Franco"
################################################################
# With explict type. #
################################################################
const INTEGER_NUMBER_2: int = -1
const REAL_NUMBER_2: float = -1.0
const LOGIC_VALUE_2: bool = true
const STRING_WITH_MESSAGE_2: String = "Hello, my name is Franco"
################################################################
# With a fixed type based in the first assignment. #
################################################################
const INTEGER_NUMBER_3 := -1
const REAL_NUMBER_3 := -1.0
const LOGIC_VALUE_3 := true
const STRING_WITH_MESSAGE_3 := "Hello, my name is Franco"
func _init():
print(INTEGER_NUMBER, ": ", typeof(INTEGER_NUMBER))
print(REAL_NUMBER, ": ", typeof(REAL_NUMBER))
print(LOGIC_VALUE, ": ", typeof(LOGIC_VALUE))
print(STRING_WITH_MESSAGE, ": ", typeof(CADEIA_CARACTERES))
print(INTEGER_NUMBER_2, ": ", typeof(INTEGER_NUMBER_2))
print(REAL_NUMBER_2, ": ", typeof(REAL_NUMBER_2))
print(LOGIC_VALUE_2, ": ", typeof(LOGIC_VALUE_2))
print(STRING_WITH_MESSAGE_2, ": ", typeof(CADEIA_CARACTERES_2))
print(INTEGER_NUMBER_3, ": ", typeof(INTEGER_NUMBER_3))
print(REAL_NUMBER_3, ": ", typeof(REAL_NUMBER_3))
print(LOGIC_VALUE_3, ": ", typeof(LOGIC_VALUE_3))
print(STRING_WITH_MESSAGE_3, ": ", typeof(CADEIA_CARACTERES_3))
#include <iostream>
#include <string>
int main()
{
const int INTEGER_NUMBER = -1;
const double REAL_NUMBER = -1.0;
const bool LOGIC_VALUE = true;
const std::string STRING_WITH_MESSAGE = "Hello, my name is Franco";
std::cout << INTEGER_NUMBER << std::endl;
std::cout << REAL_NUMBER << std::endl;
std::cout << LOGIC_VALUE << std::endl;
std::cout << STRING_WITH_MESSAGE << std::endl;
return 0;
}
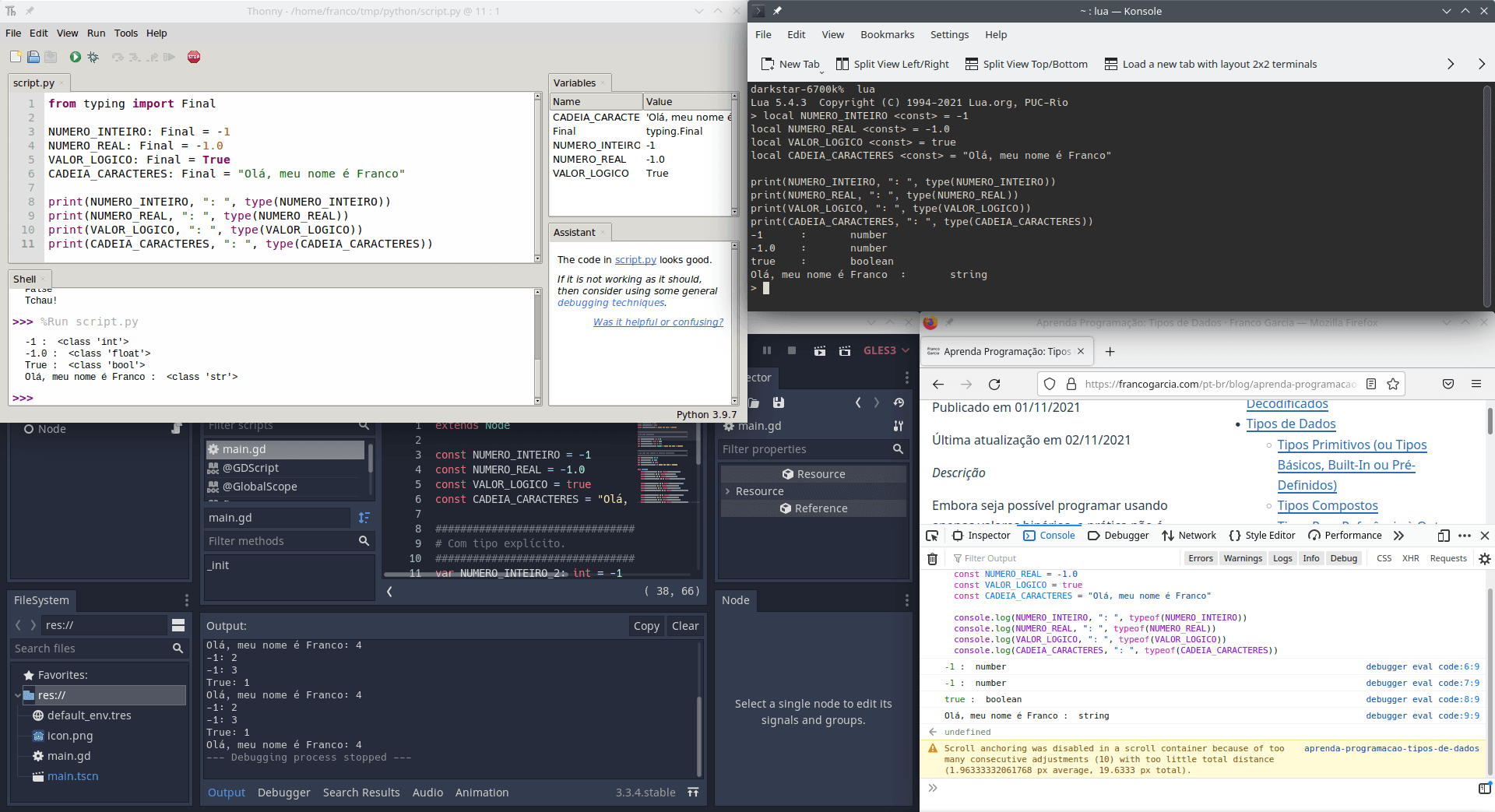
It is worth noting that there are cases in which it is possible to modify the values of constants in Python and Lua. Thus, one should take care not to modify the value by accident after the declaration.
New Items for Your Inventory
Tools:
- Inspection of variables in REPL.
Skills:
- Primary memory manipulation.
Concepts:
- Memory;
- Primary memory;
- Secondary memory;
- Variables;
- Variable declaration;
- Assignment;
- Copy;
- Swallow copy;
- Deep copy;
- Variable initialization;
- Scope;
- State;
- Constants.
Programming resources:
- Variable declaration;
- Assignment;
- Use of variables;
- Constant declaration;
- Use of constants.
Practice
Choose some data types defined in the section Practice of Data Types. Next, declare variables in a language of your choice and perform some assignments to them. Write the resulting values in the console. Although simple, this activity will exercise all the practical concepts studied up to this moment.
To complement the practice, declare some constants for each data type. Next, try to redefine the value with a new assignment. What does happen when you try to run the program?
Next Steps
With variables, you can store values in primary memory, consult them when you wish, and modify them whenever necessary. With constants, you can make it simpler to read code, and make it easier to change values later.
However, all variable values so far required to be set in the source code of the program. In other words, only people who are able to program could change the values.
To continue your software development journey, how can you allow people who, unlike you, do not yet know how to program, but wish to use programs, change values of variables?
If the output allows a system to show internal values, the input will allow external data to be processed by the program. Learning how to use input is a possible next step.
- Introduction;
- Entry point and program structure;
- Output (for console or terminal);
- Data types;
- Variables and constants;
- Input (for console or terminal);
- Arithmetic and basic Mathematics;
- Relational operations and comparisons;
- Logic operations and Boolean Algebra;
- Conditional (or selection) structures;
- Subroutines: functions and procedures;
- Repetition structures (or loops);
- Arrays, collections and data structures;
- Records (structs);
- Files and serialization (marshalling);
- Libraries;
- Command line input;
- Bitwise operations;
- Tests and debugging.
← Previous:
Learn Programming: Data Types